Dev-C, developed by Bloodshed Software, is a fully featured graphical IDE (Integrated Development Environment), which is able to create Windows or console-based C/C programs using the MinGW compiler system. MinGW (Minimalist GNU. for Windows) uses GCC (the GNU g compiler collection), which is essentially the same compiler system that is. Random numbers without the pseudo. If you really need actual random numbers and are on a Linux or BSD-like operating system, you can use the special device files /dev/random and /dev/urandom. These can be opened for reading like ordinary files, but the values read from them are a random sequence of bytes (including null characters). Here you will get program and learn how to generate random number in C and C. We can use rand function to generate random number. It is defined in stdlib.h header file. It generates a random number between 0 and RANDMAX (both inclusive). Here RANDMAX is a constant whose value depends upon the compiler you are using.
Pseudo-Random Number Generator otherwise called PRNG is a concept that involves producing a series of random numbers using mathematical formulas. Generating a random number is not as easy as it looks. When we have an algorithm and set of inputs, we get output. And if we again use the same algorithm with the same set of inputs, we get the same output and not a different one. We cannot produce a truly random number using any algorithm. But we can produce pseudo-random numbers for which the numbers are almost unpredictable that it seems like a random number.
There are several algorithms available to produce PRNG. We will be seeing a couple of basic algorithms to understand the concept and implement them using javascript.
Middle Square Method in JavaScript
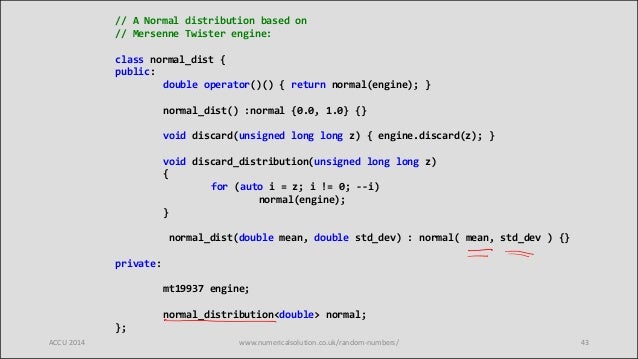
This is one of the simplest algorithms to produce a Pseudo-random number. It has the following step.
- Take a seed value (s), of fixed length/size (n), example, n = 4 and s = 1242
- Square the value of s, the resultant value will atmost be of length 2 times of n, if not padd 0 to the left of the resultant value, and letβs call it as S. Example sqr(s) = 1542564, S = 01542564
- Take the middle 4 digits from S. It is the random value obtained using the seed value s. Example, random number = 5425
- Now use this random number as the new seed value and generate the next random number by following step number 2
- If you want the number between 0 to 1, divide the resultant number by the maximum number that can be formed using n digits. For example, 5425/9999 = 0.54255425542
Output
Linear Congruential Generator in JavaScript
Linear congruential generator is a popular PRNG algorithm and it is used in lots of programming languages and systems. It is simple to implement and faster to execute. It is also a way lot better algorithm than Middle Square Method.
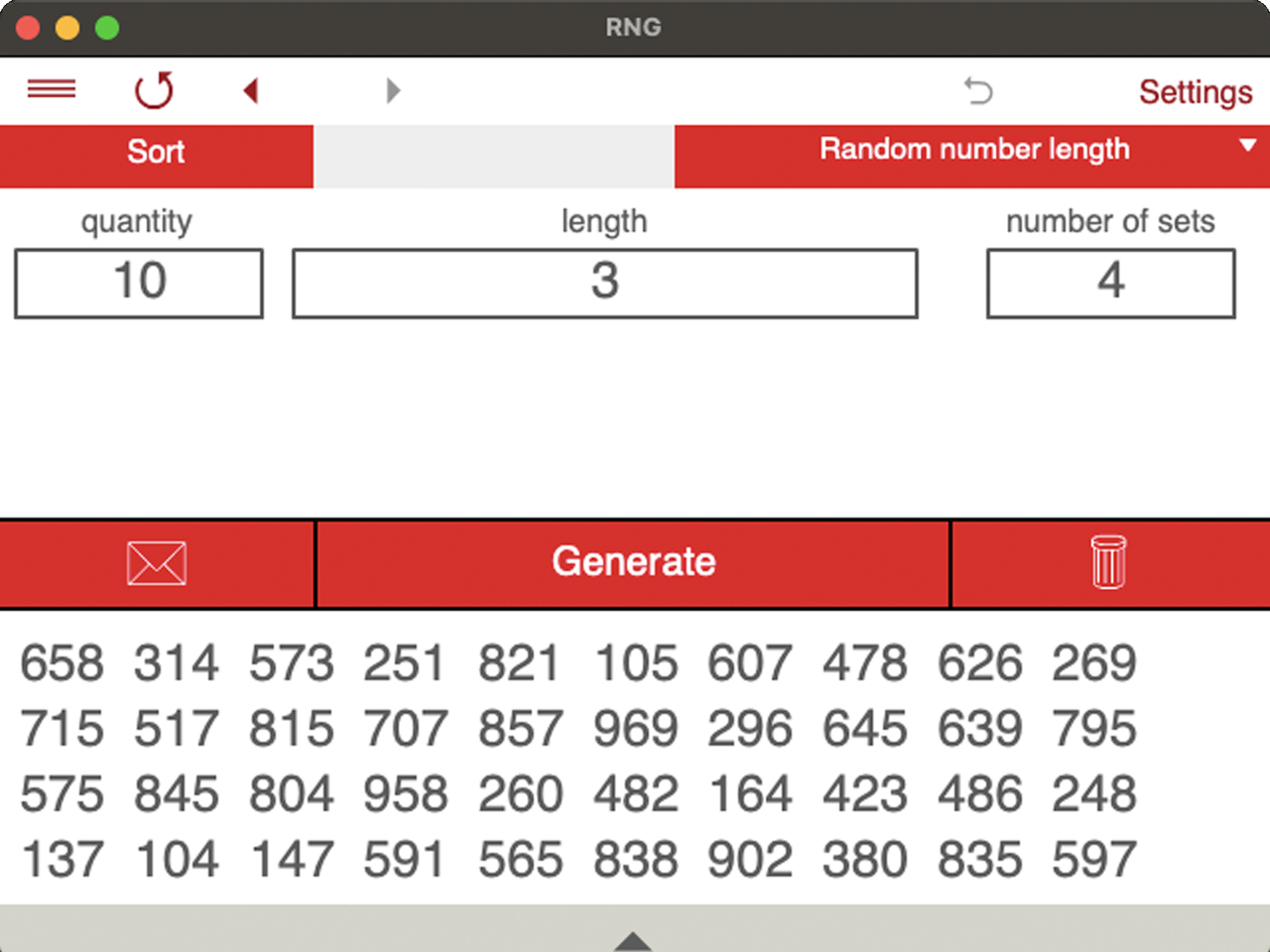
Formula :
where,
seed, 0 < seed < m is the initial value that is provided to the algorithm,
a, 0 < a < m β is the multiplier,
c, 0< c < m β is the increment,
m, 0 < m β is the modulus.
To produce a random number between 0 and 1, we divide the generated number by m. The random number generated will be used as seed value to generate next random number.
Output
How To Generate Random Number In Dev C Programming
Please post your comments, doubts, queries in the comment box. Happy Learning π
Also, read
How To Generate Random Number In Dev C++ Free
Leave a Reply
